Hey guys, in this tutorial i want to show you how to make a menu where is possible to write, using keyboard.
The menu is the most important thing and it looks like this:
PHP Code:
#include "ui_mp/menudef.h"
#define ORIGIN_TITLE 48 64
#define ORIGIN_CHOICE1 150 150
#define ORIGIN_CHOICE2 80 150
{
menuDef
{
name "keyboard"
rect 0 0 640 480
focuscolor GLOBAL_FOCUSED_COLOR
style WINDOW_STYLE_EMPTY
blurWorld 5.0
onEsc
{
close keyboard;
}
onOpen
{
}
onClose
{
}
// Gradient
itemDef
{
style WINDOW_STYLE_SHADER
//rect -107 0 554 480
rect 0 0 640 480 HORIZONTAL_ALIGN_FULLSCREEN VERTICAL_ALIGN_FULLSCREEN
background "gradient"
visible 1
decoration
}
#include "ui/bars.menu"
itemDef
{
type ITEM_TYPE_TEXT
visible 1
origin ORIGIN_TITLE
forecolor 1 1 1 1
text "Menu"
textfont UI_FONT_NORMAL
textscale GLOBAL_HEADER_SIZE
decoration
}
//This are the keys you will be abel to use in the menu
execKey "1" { play "mouse_click"; scriptMenuResponse "1"; }
execKey "2" { play "mouse_click"; scriptMenuResponse "2"; }
execKey "3" { play "mouse_click"; scriptMenuResponse "3"; }
execKey "4" { play "mouse_click"; scriptMenuResponse "4"; }
execKey "5" { play "mouse_click"; scriptMenuResponse "5"; }
execKey "6" { play "mouse_click"; scriptMenuResponse "6"; }
execKey "7" { play "mouse_click"; scriptMenuResponse "7"; }
execKey "8" { play "mouse_click"; scriptMenuResponse "8"; }
execKey "9" { play "mouse_click"; scriptMenuResponse "9"; }
execKey "0" { play "mouse_click"; scriptMenuResponse "0"; }
execKey "a" { play "mouse_click"; scriptMenuResponse "a"; }
execKey "b" { play "mouse_click"; scriptMenuResponse "b"; }
execKey "c" { play "mouse_click"; scriptMenuResponse "c"; }
execKey "d" { play "mouse_click"; scriptMenuResponse "d"; }
execKey "e" { play "mouse_click"; scriptMenuResponse "e"; }
execKey "f" { play "mouse_click"; scriptMenuResponse "f"; }
execKey "g" { play "mouse_click"; scriptMenuResponse "g"; }
execKey "h" { play "mouse_click"; scriptMenuResponse "h"; }
execKey "i" { play "mouse_click"; scriptMenuResponse "i"; }
execKey "j" { play "mouse_click"; scriptMenuResponse "j"; }
execKey "k" { play "mouse_click"; scriptMenuResponse "k"; }
execKey "l" { play "mouse_click"; scriptMenuResponse "l"; }
execKey "m" { play "mouse_click"; scriptMenuResponse "m"; }
execKey "n" { play "mouse_click"; scriptMenuResponse "n"; }
execKey "o" { play "mouse_click"; scriptMenuResponse "o"; }
execKey "p" { play "mouse_click"; scriptMenuResponse "p"; }
execKey "q" { play "mouse_click"; scriptMenuResponse "q"; }
execKey "r" { play "mouse_click"; scriptMenuResponse "r"; }
execKey "s" { play "mouse_click"; scriptMenuResponse "s"; }
execKey "t" { play "mouse_click"; scriptMenuResponse "t"; }
execKey "u" { play "mouse_click"; scriptMenuResponse "u"; }
execKey "v" { play "mouse_click"; scriptMenuResponse "v"; }
execKey "w" { play "mouse_click"; scriptMenuResponse "w"; }
execKey "x" { play "mouse_click"; scriptMenuResponse "x"; }
execKey "y" { play "mouse_click"; scriptMenuResponse "y"; }
execKey "z" { play "mouse_click"; scriptMenuResponse "z"; }
execKeyInt 32 { play "mouse_click"; scriptMenuResponse "_" }//space
execKeyInt 127 { play "mouse_click"; scriptMenuResponse "backspace" }//backspace
itemDef
{
name "typed_text"
visible 1
rect 0 0 128 24
origin ORIGIN_CHOICE1
forecolor GLOBAL_UNFOCUSED_COLOR
type ITEM_TYPE_TEXT
dvar text
textfont UI_FONT_NORMAL
textscale .50
}
itemDef
{
name "typed_text"
visible 1
rect 0 0 128 24
origin ORIGIN_CHOICE2
forecolor GLOBAL_UNFOCUSED_COLOR
type ITEM_TYPE_TEXT
text "Text: "
textfont UI_FONT_NORMAL
textscale .50
}
}
}
When you make the menu, you must define it in menus.gsc the code is:
PHP Code:
precacheMenu(game["menu_keyboard"]);
game["menu_keyboard"] = "keyboard";
So when the menu is done, you must activate him somehow, so make a button in ingame.menu for opening the keyboard.menu, and the code is:
PHP Code:
itemDef
{
name "button_keybord"
visible 1
rect 0 0 128 24
origin 80 204
forecolor GLOBAL_UNFOCUSED_COLOR
type ITEM_TYPE_BUTTON
text "6. Keyboard"
textfont UI_FONT_NORMAL
textscale GLOBAL_TEXT_SIZE
textstyle ITEM_TEXTSTYLE_SHADOWED
textaligny 20
action
{
play "mouse_click";
close ingame;
open keyboard;
}
onFocus
{
play "mouse_over";
}
}
Hmmm, the menu is done. Now we need to make the chars writing in the menu. Its working with this simple code:
PHP Code:
keyboard(response)
{
if(!isdefined(response))
return;
if(response == "backspace")//this is the backspace response so it deletes one char
{
newtext = getsubstr(self.text,0,self.text.size-1);//this deletes one char
self.text = newtext;//this defines the text without one char
self setClientCvar("text", self.text);//this show the new text in the menu
}
else
self thread char(response);
}
char(char)
{
if(self.text.size >35)return;//this 35 makes the char size
self.text += char;
self setClientCvar("text", self.text);
}
To make the script working, you must put this code on the end menus.gsc:
PHP Code:
else if(menu == game["menu_keyboard"])
maps\mp\gametypes\_keyboard::keyboard(response);
Now the keyboard is almost done. But it still don't work. TO make it working you must put this code in Callback_PlayerConnect():
PHP Code:
self.text="";
self setClientCvar("text", "");
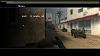
Now the keyboard is finally working. Sorry for my bad English, and i hope this tutorial will help you in the coming time.. 
The final keyboard, can be downloaded on: www.bulletbross.tk/downloads/z_keyboard.iwd